How to validate textbox to only numbers in ASP.NET?
You can validate numbers by using Regular Expressions
in ASP.NET by doing the following steps:
- In your Project, Add RegularExpressionValidator, you can add it through Toolbox.
- Click on RegularExpressionValidator, then click
F4
to open properties.
- In the properties, find
ValidationExpreesion
property write the following Expression ^\d+$
, as the below image
- Write your ValidationGroup you want for me :
OnlyNumbers
, as the below image
- Don't forget to set the
ControlToValidate
to your TextBox ID, in our case it's "txt_Numbers"
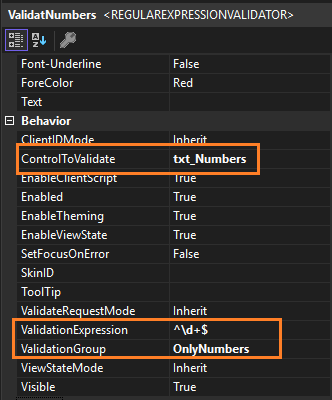
6. write your ErrorMessage you want to display, for me: Enter only numbers!
The RegularExpressionValidator should be like that:
<asp:RegularExpressionValidator ID="ValidatNumbers" runat="server" ControlToValidate="txt_Numbers"
ErrorMessage="write only numbers" ValidationExpression="^\d+$"
ValidationGroup="OnlyNumbers"></asp:RegularExpressionValidator>
- Add your
ValidationGroup
To your textbox and your button, as the image below:
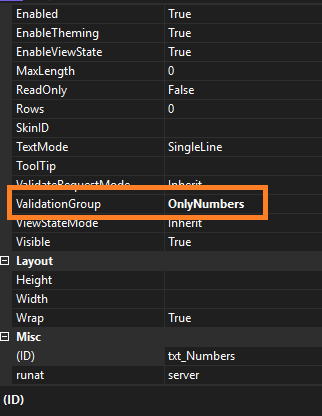
Finally the output for your textbox, button and RegularExpressionValidator:
<asp:TextBox ID="txt_Numbers" runat="server" ValidationGroup="OnlyNumbers"></asp:TextBox>
<asp:RegularExpressionValidator ID="ValidatNumbers" runat="server" ControlToValidate="txt_Numbers"
ErrorMessage="write only numbers" ValidationExpression="^\d+$"
ValidationGroup="OnlyNumbers"></asp:RegularExpressionValidator>
<asp:Button ID="btnSubmit" Text="Submit" runat="server" ValidationGroup="OnlyNumbers" />