In this post, we are gonna discuss
- Python Operators, as well as,
- Different types of operators in Python.
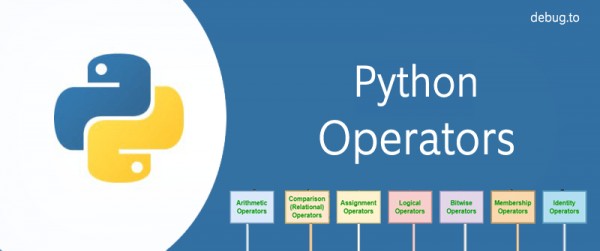
Operators in Python
- The operator is a special symbol that specifies which operations you will perform on the values of various data types.
- Python divides the operator's type into these categories:
- Arithmetic operators,
- Assignment operators,
- Comparison operators,
- Logical operators,
- Membership operator,
- Identity operators
- and Bitwise operators
Arithmetic operators
Used with numeric values to perform mathematical operations.
+ Operator (Addition)
Addition operation " add value to another value".
Ex:
x = 5
y = 6
print(x + y) #the output will be 11
- Operator (Subtraction)
Used to subtract a value from another value.
Ex:
x = 5
y = 6
print(y - x)# the output will be 1
* Operator (Multiplication)
Used to multiply two values.
Ex:
x = 5
y = 6
print(x * y)# the output will be 30
/ Operator (Division)
Used to divide two values.
Ex:
x = 30
y = 6
print(x / y) # the output will be 5
% Operator (Module)
Return the division remainder.
Ex:
x = 5
y = 2
print(x % y)# the output will be 1
** Operator (Exponentiation)
Raises the first number to the power of the second.
Ex:
x = 2
y = 5
print(x ** y)# the output will be 32 same as 2*2*2*2*2
// Operator (Floor division)
Divides and returns the integer value of the quotient. It dumps the digits after the decimal.
Ex:
x = 15
y = 2
print(x // y)# the output will be 7
Assignment operators
We will list some of the most used assignment operations:
"= " The assignment operator
Assigns the value of its right-hand operand to a variable.
Ex:
x=2
print(x)# the output will be 2
+= The addition assignment operator
Add a value to a variable in the same line.
Ex:
x=2
x+=1 # means x=x+1
print(x) # the output will be 3
- = The subtraction assignment operator
Add a value to a variable in the same line.
Ex:
x=2
x-=1 # means x=x-1
print(x) # the output will be 1
*= Operator "Multiply and Assign"
Ex:
x=2
x*=2 # means x=x*2
print(x) # the output will be 4
/= Operator "Divide and Assign"
Ex:
x=2
x/=2 # means x=x/2
print(x) # the output will be 1
%= Operator " Modulus and Assign"
Ex:
x=5
x%=3 # means x=x%3
print(x) # the output will be 2
//= Operator "Floor divide and Assign"
Ex:
x=5
x//=3 # means x=x//3
print(x) # the output will be 1
**= Operator " Exponent and Assign"
Ex:
x=5
x**=3 # means x=x**3
print(x) # the output will be 125
Logical Operators
Logical operations used to perform logical operations such as AND operation "and", OR operation "or" and the "not" negation operation.
AND Operator:
- It gives true only if the two operands is true.
- If one of the operands is false, the AND operator will evaluate it to false.
Ex:
x=5
print(x>2 and x<6)# the output will be true
or Operator:
- It gives true if one of the two operands at least is true.
- If the two operands are false, the OR operator will evaluate it to false.
Ex:
x=5
print(x>2 || x<4) # the output will be true one condition is true x>2
Negation Operator "not":
It reverses the result, and it gives true if the result is false and false if the result is true.
Ex:
x=5
print(not(x>2 or x<4)) # the output will be false
Comparison Operators
Comparison operators used to compare two values.
"==" Equal to:
Ex:
x=5
y=6
print(x == y) # the output will be false
"!=" Not Equal to:
Ex:
x=5
y=6
print(x != y) # the output will be true
">" Greater than and "<" less than
Ex:
x=5
y=6
print(x > y) # the output will be false
print(x<y) # the output will be true
">=" Greater than or Equal and "<=" Less than or Equal
Ex:
x=5
y=5
print(x >= y)# the output will be true
print(x <= y)# the output will be true
Identity Operators
- Test if the two operands share an identity.
- Compare the objects, not if they are equal, but if they are actually the same object, with the same memory location
- Python divides them into "is" and "is not" operator.
is operator
x = ["red", "yellow"]
y = ["red", "yellow"]
z = x
print(x is y) # the output will be false even if they have the same content
print(x is z) # the output will be true
is not operator
x = ["red", "yellow"]
y = ["red", "yellow"]
z = x
print(x is not y) # the output will be true even if they have the same content
print(x is not z) # the output will be false
Membership Operators
- These Python operators check if a value is a member of a sequence " string, list, tuple".
- There are two memberships operator in Python "in" and "not in".
in operator
- Checks if a value "sequence of value" is present in the sequence.
- It returns true if it found the value in the sequence otherwise it return false.
Ex:
x = ["red", "yellow"]
print("red" in x)#the output will be true as the sequence red is a member of the list.
not in operator
- Checks if a value "sequence of value" is not present in the sequence.
- It returns true if it didn't find the value in the sequence otherwise it returns false.
Ex:
x = ["red", "yellow"]
print("red" not in x)#the output will be false as the sequence red is a member of the list.
Bitwise operators
Used in the comparison of binary numbers.
& operator "and"
sets the bit to 1 if the two bits are 1 otherwise if one bit at least 0 then it sets the bit to 0
Ex:
print(2&3) #the output will be 2
since the binary for 2 is 10 and the binary for 3 is 11 then when " and " the bits of (2&3) it will be (10 &11) =(10) the bits of 2 so the result will be 2
| operator "or"
Sets the bit to 1 if one of the two bits at least is 1 otherwise if the two bits is 0 then it sets the bit to 0
Ex:
print(2|3) #the output will be 3
since the binary for 2 is 10 and the binary for 3 is 11 then when " or " the bits of (2|3) it will be (10 |11) =(11) the bits of 3 so the result will be 3.
^ operator "xor:exclusive or"
sets the bit to 1 if only one of the two bits is 1 otherwise it will be 0.
Ex:
print(2^3) #the output will be 1
since the binary for 2 is 10 and the binary for 3 is 11 then when " xor " the bits of (2^3) it will be (10 ^11) =(01) the bits of 1 so the result will be 1.
~ operator "binary one's complement"
It inverts all the bits 1 to 0 and 0 to 1.
Ex:
print(~2) #the output will be -3
since the binary for 2 is 00000010 when " ~" the bits of (~2) it will be (11111101) the bits of -3 so the result will be -3.
<< operator "binary left shift"
x<<y It shifts the bits of the first operand x to the left numbers of placing depending on the second operand value y.
Ex:
print(2<<2) #the output will be 8
Since the binary for 2 is 00000010 when " <<" the bits of (<<<2) two places:
The second operand value here is 2" it will be (1000) the bits of 8. so the result will be 8.
>> operator "binary right shift"
x>>y It shifts the bits of the first operand x to the right numbers of placing depending on the second operand value y.
Ex:
print(3<<2) #the output will be 0
since the binary for 3 is 11 when " >>" the bits of (>>3) two places :the second operand value here is 2" it will be (00) the bits of 0 so the result will be 0.
Conclusion
in this post we discussed Python operators:
- Arithmetic operators,
- Assignment operators,
- Comparison operators,
- Logical operators,
- Membership operator,
- Identity operators
- and Bitwise operators
See also