In the previous post, we explained String in Python. In this post, we are going to learn the best ways of string concatenation in Python.
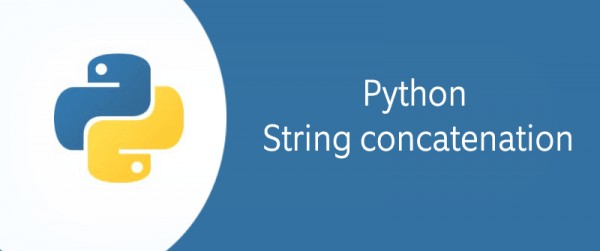
Python represents "string" as a text, it doesn't have a "character" data type.

What is string concatenation?
In Python, there are a few ways to concatenate or combine strings, as we know everything in Python is an object, so the new string is a string object.
String concatenation in Python:
To concatenate string in Python, you can use the following:
The + operator
- Concatenate one or more string.
- once the string object created, it can't be changed. (this means immutable)
- It is recommended for a few strings.
Ex:
firstName="Hamza "
lastname="Mohammed"
print(firstName+lastname)# the output will be Hamza Mohammed
+= concatenate operator
Besides the + operator, we can use the += operator to concatenate string in Python.
Ex:
firstName="Hamza "
lastname="Mohammed"
firstName+=lastname
print(firstName)# the output will be Hamza Mohammed
join() method
- It is used with iterators.
- It is recommended in case you have many strings.
Ex:
colorLists=['Red', 'Yellow', 'Black']
str_concat = ", ".join(colorLists)
print(str_concat)
Conclusion
In this post, we have explained three-way to concatenate strings in Python.
See Also