In this post, we're gonna learn how to write the different types of IF Conditions in
You may be also interested to check Loops in Programming languages
Before we getting started, let's first know more about Conditions
What're Conditions?
- Conditions are a decision-making statement.
- Perform different actions depending on a boolean condition that may be true or false.
Conditions Type
- If statement: is used to specify a block of code to be executed, if a specified condition is true.
- If - else statement: is used to specify a new condition to test, if the first condition is false then it will be redirected to the else part.
- Logical conditions: Are features of programming languages "conditional statement", we will list them below:-
- Less than: a < b
- Less than or equal to: a <= b
- Greater than: a > b
- Greater than or equal to: a >= b
- Equal to a == b
- Not Equal to: a != b
Condition In C#
- IF Statement.
- IF-ELSE statement.
- ELSE-IF Statement.
C# If statement
- Use the if statement to specify a block of C# code to be executed if a condition is True.
- The syntax of if statement in C# is:
if (condition)
{
// block of code to be executed if the condition is True
}
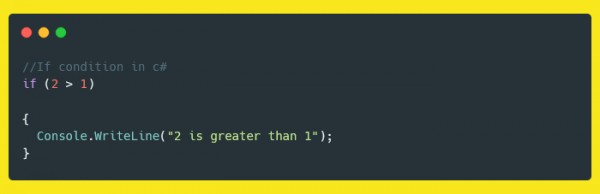
C# If – else Statement
- An if statement can be followed by an optional else statement, which executes when the first condition is FALSE.
- The syntax of If – else in C# is:
if (condition)
{
// block of code to be executed if the condition is True
}
Else
{
// block of code to be executed if the condition is True
}
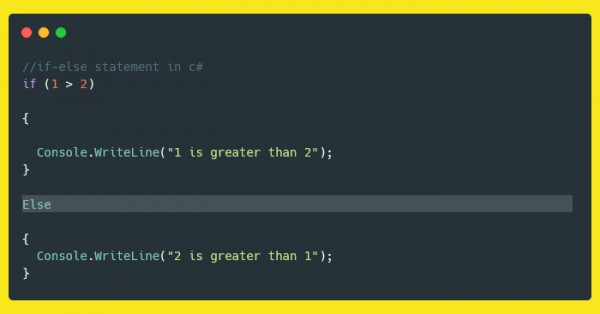
C# Else if Statement
- Use the else if statement to specify a new condition if the first condition is False.
- The syntax of else if statement in C# is
if (condition1)
{
// block of code to be executed if condition1 is True
}
else if (condition2)
{
// block of code to be executed if the condition1 is false and condition2 is True
}
else
{
// block of code to be executed if the condition1 is false and condition2 is False
}
Conditions In Python
- IF Statement
- IF-ELSE Statement
- ELIF Statement
Python If Statement
- Use the if statement to specify a block of C# code to be executed if a condition is True.
- (white space at the beginning of a line) to define the scope in the code
- The syntax of if statement in python is
if condition
#block of code to be executed if the condition is True
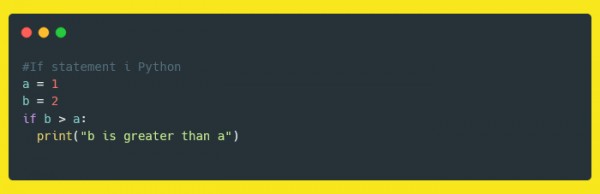
Python (If–else) Statement
- An if statement can be followed by an optional else statement, which executes when the first condition is FALSE.
- (white space at the beginning of a line) to define the scope in the code of if and else
- The syntax of If – else in Python is:
if condition
#block of code to be executed if the condition is True
else
# block of code to be executed if the condition is False
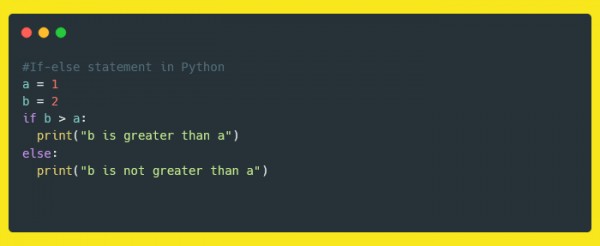
Python Elif statement
- The elif keyword in pythons is the way of saying "if the previous conditions were not true, then try this condition".
- Also, make sure that the white space at the beginning of a line is to define the scope in the code of if and Elif.
- The syntax of If – else in Python is:
if condition
#block of code to be executed if the condition is True
Elif condition
# block of code to be executed if the condition is False
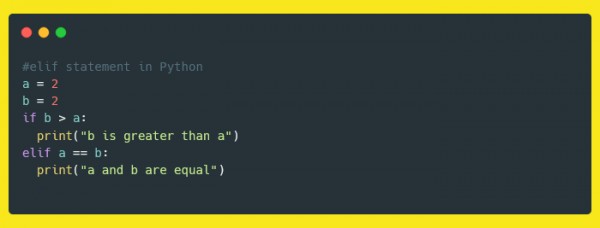
Conditions In Java
- IF Statement
- IF-ELSE Statement
- ELSE-IF Statement
Java If Statement
- Use the if statement to specify a block of C# code to be executed if a condition is True.
- The syntax of if statement in java is:
if(condition) {
// block of code to be executed if the condition is True
}
Java (If-else) Statement
- An if statement can be followed by an optional else statement, which executes when the first condition is FALSE.
- The syntax of If – else in C# is
if (condition)
{
// block of code to be executed if the condition is True
}
else
{
//block of code to be executed if the condition is false
}
Java else if Statement
- Like other programming languages the else if statement in java is to specify a new condition if the first condition is False.
- The syntax of else if statement in Java is
if (condition1)
{
// block of code to be executed if condition1 is True
}
else if (condition2)
{
// block of code to be executed if the condition1 is false and condition2 is true
}
else
{
// block of code to be executed if the condition1 is false and condition2 is False
}
Conclusion
In this post, we have learned how to write different Conditions in C#, Python, and Java programming language.
See Also